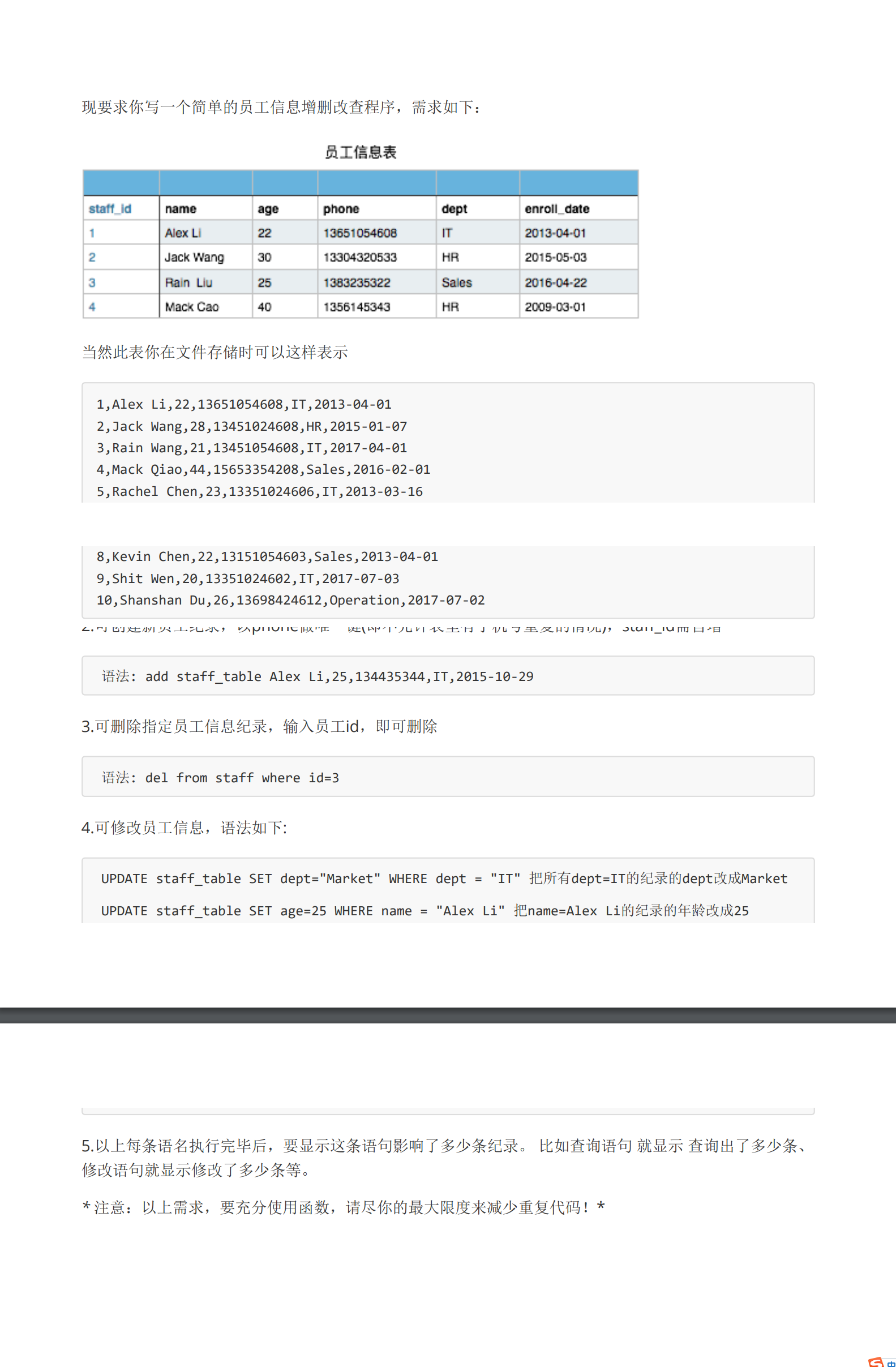
# -*- coding:utf-8 -*- # author: heimu import os staff_msg = '''1,Alex Li,22,13651054608,IT,2013-04-01 2,Jack Wang,28,13451024608,HR,2015-01-07 3,Rain Wang,21,13451054608,IT,2017-04-01 4,Mack Qiao,44,15653354208,Sales,2016-02-01 5,Rachel Chen,23,13351024606,IT,2013-03-16 6,Eric Liu,19,18531054602,Marketing,2012-12-01 7,Chao Zhang,21,13235324334,Administration,2011-08-08 8,Kevin Chen,22,13151054603,Sales,2013-04-01 9,Shit Wen,20,13351024602,IT,2017-07-03 10,Shanshan Du,26,13698424612,Operation,2017-07-02 ''' def init_staff_msg(): ''' 初始化员工信息表 :return: ''' with open('staff_msg.txt','w') as f: f.writelines(staff_msg) def print_staff_msg(): ''' 打印出员工信息表 :return: ''' print('-------------------员工信息表-----------------') with open('staff_msg.txt','r') as f: for line in f: print(line) def inquire_msg(): ''' 模糊查询员工信息 :return: ''' msg = ''' 请按照如下样例进行查询: 1. find name,age from staff_table where age > 22 2. find * from staff_table where dept = "IT" 3. find * from staff_table where enroll_date like "2013" ''' update_staff_msg = [] # 存储满足查询条件的员工信息 while True: print(msg) user_input = input('请输入查询命令>>>:').strip().split(' ') # 按照空格分割,得到一个列表 if user_input[5] == 'age': # 按照条件1查询 with open('staff_msg.txt','r') as f: for line in f: staff_msg_list = line.strip().split(',') # 将字符串按照','分割成一个列表 if staff_msg_list[2] > user_input[7]: update_staff_msg.append(staff_msg_list) # 将满足条件的员工信息加入到列表 break elif user_input[5] == 'dept': # 按照条件2查询 with open('staff_msg.txt','r') as f: for line in f: staff_msg_list = line.strip().split(',') if staff_msg_list[4] == eval(user_input[7]): update_staff_msg.append(staff_msg_list) break elif user_input[5] == 'enroll_date': # 按照条件3查询 with open('staff_msg.txt','r') as f: for line in f: staff_msg_list = line.strip().split(',') if staff_msg_list[5].split('-')[0] == eval(user_input[7]): update_staff_msg.append(staff_msg_list) break else: print("输入错误,请重新输入。") print("满足查询条件的员工信息如下,人数为:\033[31;1m%d\033[0m。" % len(update_staff_msg)) [print(update_staff_msg[i]) for i in range(len(update_staff_msg))] def add_new_staff(): ''' 创建新员工记录 :return: ''' msg = ''' 请按照如下样例创建新员工记录: add staff_table Alex Li,25,134435344,IT,2015-10-29 ''' while True: print(msg) user_input = input("请输入命令>>>:").strip().split(',') if user_input[0].split(' ')[0] == 'add': # 输入格式正确 user_input[0] = ' '.join(user_input[0].split(' ')[2:]) # add staff_table Alex Li ---> Alex Li with open('staff_msg.txt','r+') as f: staff_phone = [] # 存储员工信息的电话号码phone for line in f: staff_phone.append(line.strip().split(',')[3]) if user_input[2] in staff_phone: print("这条记录已经存在。") else: new_staff_index = str(len(staff_phone) + 1) # 得到新添加员工的索引 user_input.insert(0,new_staff_index) # 将索引值插入到最前面 user_input = ','.join(user_input) # 列表-->字符串 f.write(user_input) f.write('\n') print("新员工记录添加完成。") break else: print("输入错误,请重新输入。") def delete_staff(): ''' 删除员工信息 :return: ''' msg = ''' 请按照如下样例删除员工信息: del from staff where id=3 ''' while True: print(msg) user_input = input('请输入命令>>>:').strip().split(' ') if user_input[0] == 'del': file_old = open('staff_msg.txt','r+') file_new = open('staff_msg_new.txt','w') for line in file_old: line_split = line.strip().split(',') if user_input[4].split('=')[1] != line_split[0]: # 不符合条件的员工信息保存下来,写入新的文件 file_new.write(line) file_new.close() file_old.close() os.remove('staff_msg.txt') # 删除旧文件 os.rename('staff_msg_new.txt','staff_msg.txt') # 重命名新文件 print("员工信息删除成功。") break else: print("输入错误,请重新输入") def update_staff_msg(): ''' 更新员工信息 :return: ''' msg = ''' 请按照如下样例更新员工信息: UPDATE staff_table SET dept="Market" WHERE dept="IT" UPDATE staff_table SET age=25 WHERE name="Alex Li" ''' while True: print(msg) user_input_raw = input('请输入命令>>>:').strip() user_input = user_input_raw.split(' ') if user_input[0].lower() == 'update': count = 0 # 计数,记录的改动次数 file_old = open('staff_msg.txt','r+') file_new = open('staff_msg_new.txt','w+') update_msg_new = [] # 存储更新后的员工信息 update_msg_old = [] # 存储更新前的员工信息 for line in file_old: line = line.strip().split(',') # 字符串-->列表 if user_input[5].split('=')[0] == 'dept': # 按照样例1更新员工信息 if line[4] == eval(user_input[5].split('=')[1]): update_msg_old.append(','.join(line)) line[4] = eval(user_input[3].split('=')[1]) line = ','.join(line) count+=1 update_msg_new.append(line) else: line = ','.join(line) elif user_input[5].split('=')[0] == 'name': # 按照样例2更新员工信息 if line[1] == eval(user_input_raw.split('=')[2]): update_msg_old.append(','.join(line)) line[2] = user_input[3].split('=')[1] line = ','.join(line) count+=1 update_msg_new.append(line) else: line = ','.join(line) else: pass file_new.write(line) file_new.write('\n') print("您总共修改了\033[31;1m%d\033[0m条记录" % count) print("修改前的\033[31;1m%d\033[0m个员工信息" % count) [print(update_msg_old[i]) for i in range(len(update_msg_old))] print("修改后的\033[31;1m%d\033[0m个员工信息" % count) [print(update_msg_new[i]) for i in range(len(update_msg_new))] file_old.close() file_new.close() os.remove('staff_msg.txt') os.rename('staff_msg_new.txt','staff_msg.txt') break else: print("输入错误,请重新输入。") def main(): ''' 主函数 :return: ''' msg = ''' 1、查询员工信息 2、添加新员工信息 3、删除员工信息 4、更改员工信息 5、打印所有员工信息 6、退出程序 ''' init_staff_msg() while True: print(msg) user_choose = input("请输入你的选择>>>") if user_choose == '1': inquire_msg() elif user_choose == '2': add_new_staff() elif user_choose == '3': delete_staff() elif user_choose == '4': update_staff_msg() elif user_choose == '5': print_staff_msg() elif user_choose == '6': exit("程序结束!!!") else: print("您的选择有误,请重新输入") if __name__ == '__main__': main()
---
转载请注明本文标题和链接:《Python第十七天 :员工信息表》
发表评论